If you Google "build test release npm module" this is the top result. Cool, huh?
⚠️ This post is over two years old and may contain some outdated technical information. Please proceed with caution!
I had never actually built or released a node module before @whitep4nth3r/random-code, and this blog post by Alec Mather was super helpful in understanding the concepts. The most important thing I learned was that if we want to write the code for the node module in ES6, we need a way to transpile the code from ES6 to ES5 so that it’s compatible with anyone’s code.
I don’t intend to recreate the tutorial in this post, but instead, write out the process for future me. I can see myself harnessing the power of building and releasing node modules much more as time goes on!
If you’d prefer to watch this process from start to finish, check out this five-minute quick fire-video on YouTube that shows me learning how to create my first node module and publish it to npm — live on Twitch!
Click the video above to play
Let’s go through step by step how to create, build and publish a package to npm that’s written in ES6.
Prerequisites
Ensure you’ve installed Node.js and npm on your machine.
Create an account on npm
You’ll need this to be able to publish your package. Sign up here.
Login to npm via your terminal
Run npm login
in your terminal and enter your username, password and email. This will ensure you can publish your package later via the CLI.
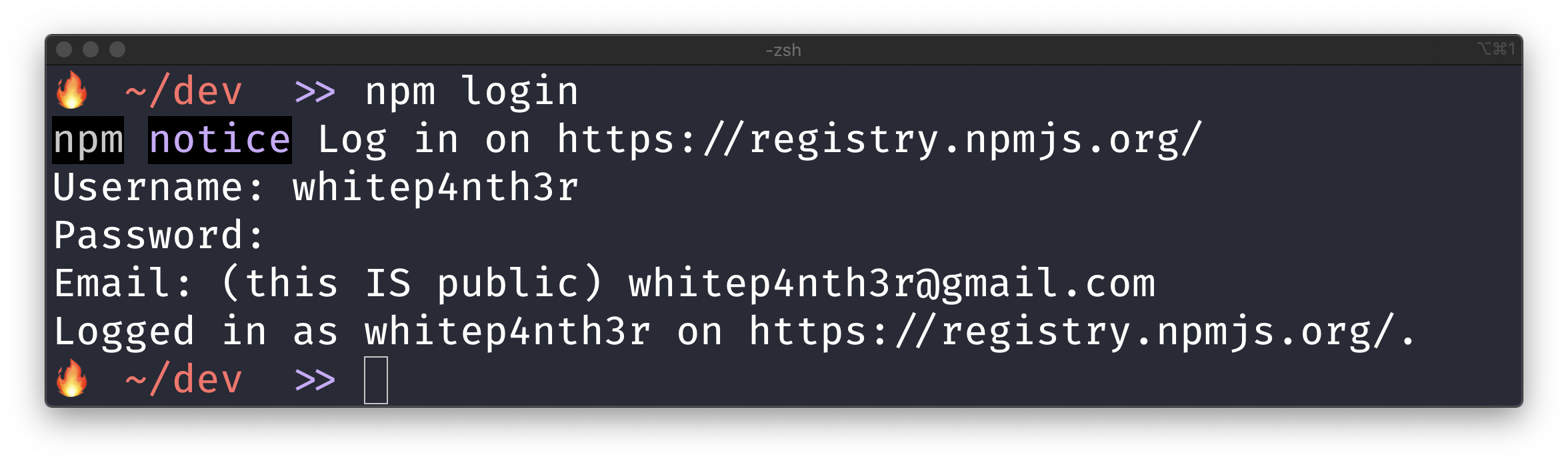
Set up your project
Create a new directory for your project that will contain the code for your npm package. Navigate to that directory. For unscoped modules (without the @scope-name prefix), run npm init
. This will create the package.json file for your project.
For my first node module, I decided to create a scoped public package, so that it would include my brand name. To initialise a scoped module, run npm init --scope=@scope-name
. Follow the instructions in the terminal to configure your project. Read more about scoped public packages here.
mkdir my-new-npm-package
cd my-new-npm-package
npm init
# or for scoped packages
npm init --scope=@scope-name
Here’s the package.json file that was created via the CLI for @whitep4nth3r/random-code.
{
"name": "@whitep4nth3r/random-code",
"version": "1.0.0",
"description": "Need some code for your project? We've got you covered. Choose your language. Choose how much code. BÄM! You got code.",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [
"random"
],
"author": "whitep4nth3r",
"license": "MIT"
}
At the root of your new project, create the following directories:
src
— this is where we’ll store our ES6 code filesdist
— this is where we’ll store the transpiled ES5 code
Inside the src
folder, create an index.js
file. This is the file that will export all of your ES6 code from this directory.
Finally, at the root of the project, create an index.js
file, and add this line of code.
module.exports = require("./dist");
This is the entry point to our application, as specified in the main
field in the package.json file. This instructs whatever code is consuming the node module to load all of the contents of the dist
directory, where our transpiled ES5 code will be.
Here’s how your project structure should look so far:
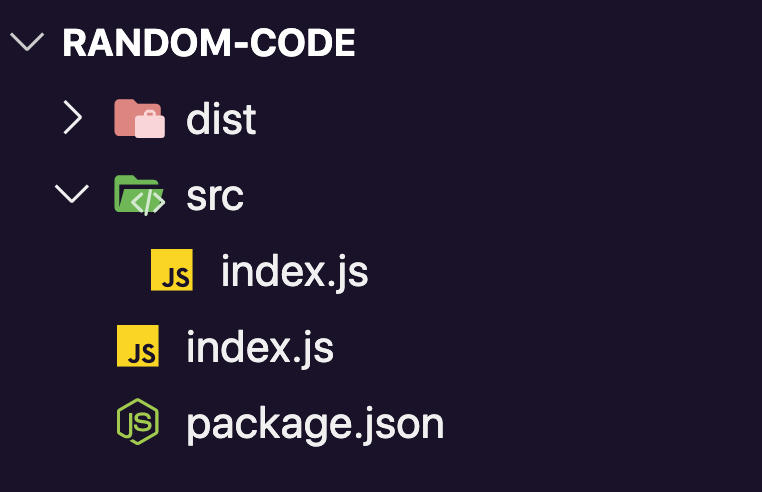
Write some ES6 code in the src directory
I can’t help you with this bit — but go wild! Ensure that each function you want to export from the src
directory in index.js
is prefixed with export
. Check out the equivalent file for the random-code node module on GitHub.
At this point you’ll probably want to create a git repository for your node module package to ensure your hard work is version-controlled.
Transpile your ES6 code to ES5 using babel
We need to install two dev dependencies to transpile the ES6 code to ES5.
In your terminal, run:
npm install --save-dev @babel/cli @babel/core @babel/preset-env
Next, at the root of your project, add a .babelrc
file, and add the following lines:
{
"presets": ["@babel/preset-env"]
}
Next, add the following build command to your package.json file.
"scripts": {
"build": "babel src -d dist"
}
Now, when you run npm run build
, babel will transpile all of the code from the src
directory from ES6 to ES5, and place it in dist
. Make sure you run npm run build
each time you want to test your code locally in a different directory or project.
Wait, I can test my npm package locally? You sure can! Here’s how.
Test your node module before you publish
We can use npm link
to test out the functionality of an npm package without publishing it.
In your node module project directory, run the following command:
npm link
In an existing project directory, or a new directory where you wish to test this npm package (assuming the project already has a package.json), run the following command:
npm link my-new-npm-package
# or for scoped projects
npm link @scope-name/my-new-npm-package
You can now import the node module as you would if it was published to npm, for example:
import { getLanguages, generateRandomCode } from "@whitep4nth3r/random-code";
Publish your new node module
When you’ve tested your new node module and you’re happy with the results, it’s ready to be published!
At the root of your npm package directory, make sure you are logged into npm via the CLI as described above, and run the following command in your terminal:
# for unscoped packages
npm publish
# for scoped packages
npm publish --access public
Ensure that you increment the version number in package.json each time you want to publish.
And there you have it!
View the @whitep4nther/random-code node module on npm.
The npm ecosystem is a great way to distribute useful blocks of reusable code. Through learning how to build and publish packages to npm, I feel like I’ve really levelled-up my web dev skills. Good luck in creating your first npm package!
If you've tried out the random-code package on npm, I'd love to hear about it! Come and say hi on Twitch!
And remember, build stuff, learn things and love what you do.
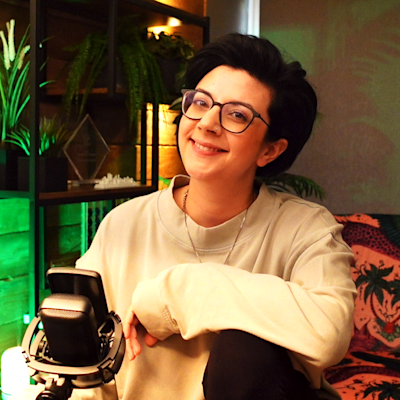
Salma Alam-Naylor
I'm a live streamer, software engineer, and developer educator. I help developers build cool stuff with blog posts, videos, live coding and open source projects.